Inharitance with Java
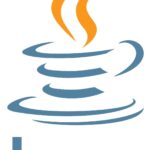
Are you a functional programmer who’s interested in learning about inheritance in Java?
Inheritance is a powerful concept that lets you reuse code with shared attributes and behaviors,
making your code more modular and maintainable. Even if you’re used to thinking in terms of
functions and data structures, understanding inheritance in Java can help you write more
efficient and organized code. In this tutorial, we’ll explore the Animal-Dog example and show you
how to use inheritance to create better code in Java.
Inheritance
Inheritance is a mechanism in object-oriented programming that allows a new class to be based
on an existing class. The new class, known as the subclass , inherits all the properties and
behaviors of the existing class, known as the superclass. The subclass can then add its own
properties and behaviors or override existing ones.
Inheritance is implemented using theextendskeyword. To create a subclass, you specify the
name of the superclass followed by the extends keyword and then the name of the subclass.
Here is an example:
superclass:
public class Animal {
public void eat() {
System.out.println("I am eating");
}
}
subclass:
public class Dog extends Animal {
public void bark() {
System.out.println("Woof!");
}
}
In this example the Dog class extends the Animal class. This means that the Dog class inherits
the eat() method from the Animal class. The Dog class also has its own bark() method.
public class Main {
public static void main(String[] args) {
Animal a = new Animal();
a.eat();// i am eating
Dog d = new Dog();
d.eat();// i am eating
d.bark();// woof
}
}
The animal object canonlycall the eat() method,while the dog object can call both the eat() and
bark() methods.
Constructors in inheritance
A constructor is a special method that is called when an object of a class is created. When a
subclass is created, its constructor must call the constructor of its superclass. This can be
achieved using the super() method, which calls the constructor of the superclass. Here’s an
example:
public class Animal {
public Animal() {
System.out.println("Animal constructor");
}
}
public class Dog extends Animal {
public Dog() {
super(); //this will be called even if we remove the Dog constructor
}
}
public class Main {
public static void main(String[] args) {
Animal a = new Animal();//Animal constructor
a.eat(); // i am eating
Dog d = new Dog();//Animal constructor
d.eat(); // i am eating
d.bark(); // woof
}
}
When a subclass is created, its constructor automatically calls the constructor of its superclass,
even if you don’t explicitly use the super() method in the subclass construct
Method Overriding
Method overriding is a technique that allows a subclass to provide its own implementation of a
method that is already defined in its superclass. This is useful when the behavior of a method
needs to be modified in the subclass.
public class Animal {
public Animal() {
System.out.println("Animal constructor");
}
public void eat(){
System.out.println(" i am eating");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("woof");
}
@Override
public void eat() {
System.out.println("im a dog, and i am eating like a dog");
}
}
public class Main {
public static void main(String[] args) {
Animal a = new Animal();//Animal constructor
a.eat();// i am eating
Dog d = new Dog();//Animal constructor
d.eat();//im a dog, and i am eating like a dog
d.bark();// woof
}
}
Final Classes and Methods
You can use thefinalkeyword to prevent a class frombeing subclassed or a method from being
overridden. This can be useful when you want to ensure that a certain behavior or
implementation cannot be modified by subclasses. For example:
public final class Animal {
public final void eat() {
System.out.println("I am eating");
}
}
public class Dog extends Animal{
public void bark() {
System.out.println("I am barking");
}
}
@override
public void eat(){
System.out.println("I am eating");
}
A Visualization of the Animal-Dog example:
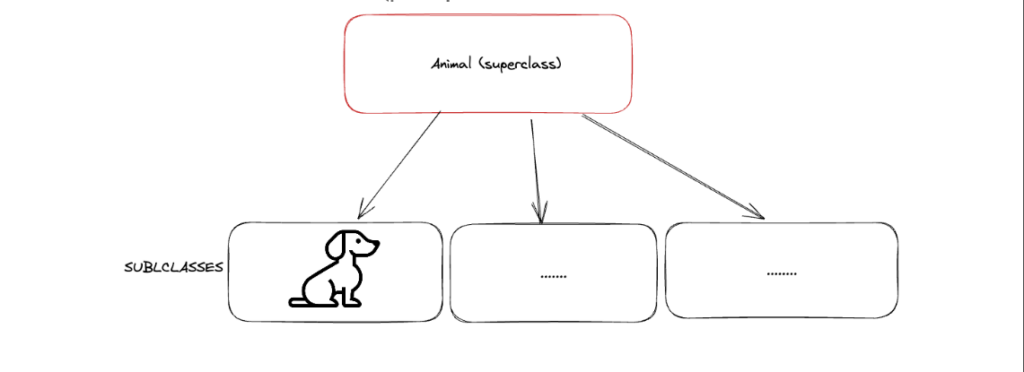
Conclusion
Inheritance is a powerful feature of object-oriented programming that allows you to create new
classes based on existing ones. It enables you to reuse code and create a hierarchical structure
of classes that share common characteristics.
Inheritance is achieved using theextendskeywordto create a subclass that inherits properties
and methods from a superclass. The subclass can then add new properties and methods, or
overrideexisting ones.
When you create a subclass, its constructor automatically calls the constructor of its
superclass, even if you don’t explicitly use the super() method in the subclass constructor. If you
want to call a specific constructor of the superclass, you can use the super() method with the
appropriate arguments.
Halil Kömürcü
Designbees Praktikant – 2023